# BLE SDK for Android
# Introduction
The NBIoTBleKit SDK allows you to build your own APP for controlling segway vehicles via Bluetooth quickly.
# Get Started
# Step 1: Get an SDK key and operator code
First of all, you should get the SDK key and operator code. You’ll be able to find your key in the backend following these steps.
Click the icon to bring up the drop-down menu, then click the Basic information item.
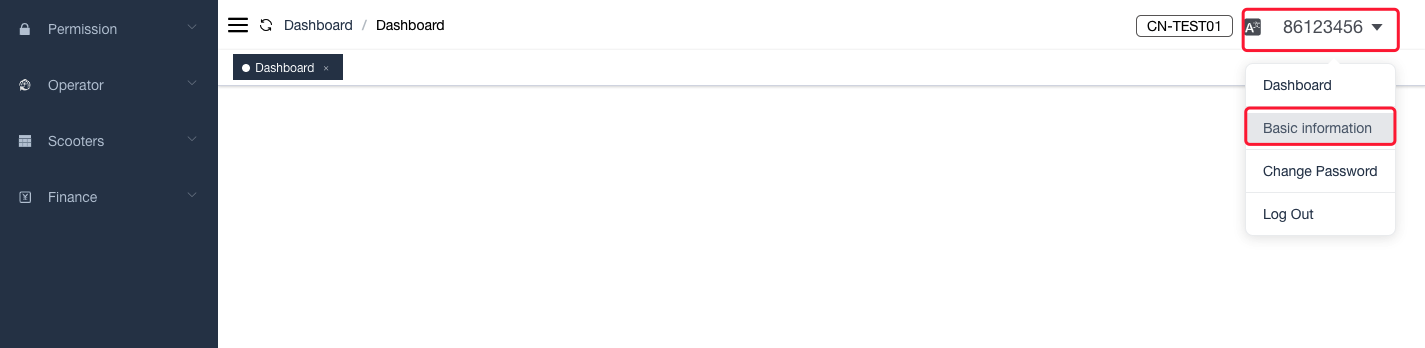
Scroll to the bottom, you can see the View button following the Bluetooth SDK key box.
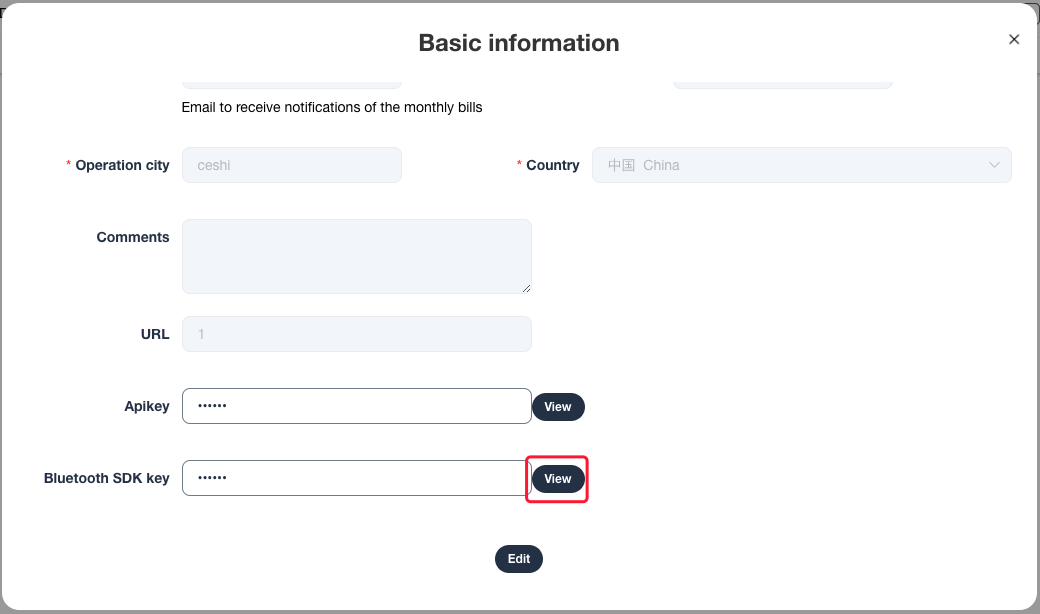
Click the View button and type your password to confirm.
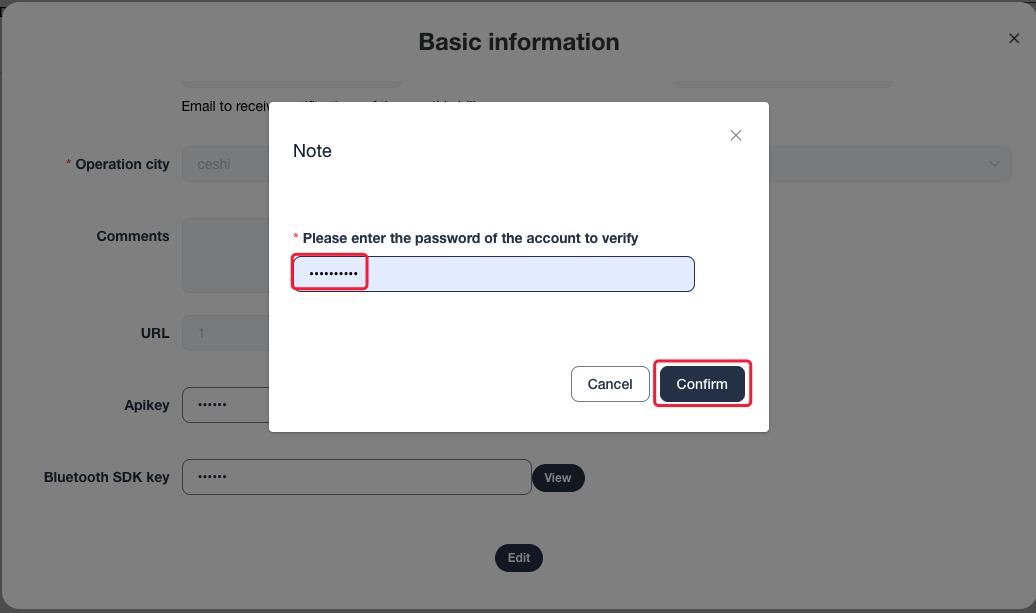
Good job, now you can see your key, and you can copy that through a quick button.
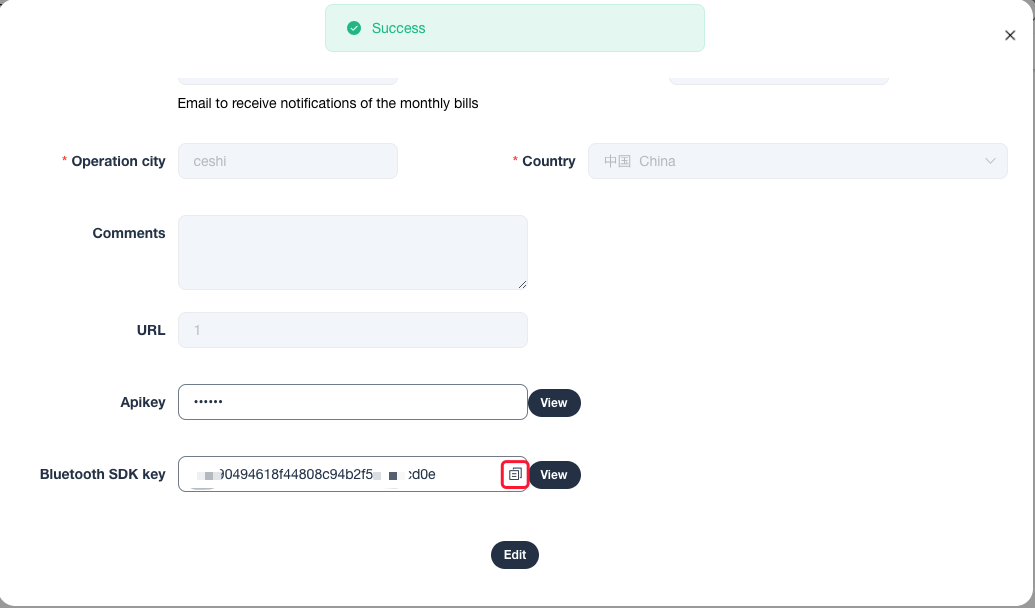
Done, any problem please contact us.
# Step 2: Set up the development environment
Android Studio is required. You can download and install it if not.
# Step 3: Create your project
Open Android Studio, click the button of Create New Project in the window of Welcome to Android Studio.
In the New Project window, under the Phone and Tablet category, select the Activity, and then click Next.
Complete the BLE Activity form:
Set Minimum SDK to an Android SDK version that is supported by your test device.
When you finish creating your project, Android Studio will start Gradle and build the project. This may take some time.
For more information about creating a project, see Create an Android Project .
# Step 4: Dependencies Files Import
click here to download jar and so files
Unpack the compressed package and then copy the file to the app libs directory as follows;
2.Open your app-level build.gradle file and add the following code;s
android {
defaultConfig {
ndk {
abiFilters "armeabi-v7a", "arm64-v8a", "x86", 'x86_64'
}
}
sourceSets {
main {
jniLibs.srcDirs = ['libs']
}
}
}
- Click Sync button to sync the project.
# Dev Guides
You can click code samples to download sample project.
# Step 1: Configure the permissions
Configure the permissions in your AndroidManifest.xml
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
# Step 2: Adding the SDK key and operator code to your APP
Add the following code to your application file. In the following examples, replace secretKey
with your SDK key, and operatorCode
with your operator code.and in the debug mode.you can see detail log msg.
public class IoTManagerApplication extends Application {
@Override
public void onCreate() {
NBIotBle.getInstance().init("secretKey", "operatorCode", isDebug);
}
}
# Vehicle API Guide
# Init
WARNING
Before calling this method, you need to init the NBIotBle in your application, otherwise you will not get the relative permission.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//init BluetoothKit
BluetoothKit bluetoothKit = new BluetoothKit();
bluetoothKit.init(this);
}
# Connect
Connect the device with the MAC Address,deviceKey and deviceIMEI, make sure the vehicle is not connected to another bluetooth device before connecting.
private void connect() {
bluetoothKit.connect("deviceMac", "deviceKey","deviceIMEI" new OnConnectionStateChangeListener() {
@Override
public void onConnectionStateChange(int state) {
switch (state) {
case ConnectionState.STATE_CONNECTED:
Log.d("NBBleSDK","Connected");
break;
case ConnectionState.STATE_DISCONNECTED:
Log.d("NBBleSDK","Disconnected");
break;
default:
Log.d("NBBleSDK","ConnectFailed");
break;
}
}
});
}
# Disconnect
Disconnect from a device
if (bluetoothKit != null) {
bluetoothKit.disConnect();
}
# Unlock
bluetoothKit.unLock(new OnUnlockListener() {
@Override
public void onUnlockSuccess() {
Log.d("bluetoothKit","UnlockSuccess");
}
@Override
public void onUnlockFail(int code, String msg) {
Log.e("bluetoothKit","UnlockFail");
}
});
# Lock
bluetoothKit.lock(new OnLockListener() {
@Override
public void onLockSuccess() {
Log.d("bluetoothKit","LockSuccess");
}
@Override
public void onLockFail(int code, String msg) {
Log.e("bluetoothKit","LockFail");
}
});
# Query IoT information
bluetoothKit.queryIoTInformation(new OnQueryIoTInfoListener() {
@Override
public void onQueryIoTInfoSuccess(QueryIoTInfomation queryIoTInfomation) {
Log.d("bluetoothKit","QueryIoTInfoSuccess");
}
@Override
public void onQueryIoTInfoFail(int code, String msg) {
Log.e("bluetoothKit","QueryIoTInfoFail");
}
});
attached:'QueryIoTInformation'
field | type | description | note |
---|---|---|---|
voltage | int | current voltage of IoT, unit: mV | supported from v1.1.0 |
isLocked | boolean | true = locked,false = unlocked | supported from v1.1.0 |
majorVersionNumber | int | major versionNumber | |
minorVersionNumber | int | minor versionNumber | |
updateTimes | int | IoT device software version update times | supported from v1.1.0 |
highBatteryVoltage | int | high battery voltage | deprecated from v1.1.0 |
lowBatteryVoltage | int | low battery voltage | deprecated from v1.1.0 |
powerStatus | int | power status | deprecated from v1.1.0 |
reserve | int | reserve | deprecated from v1.1.0 |
versionRevisions | int | use `updateTimes` instead | deprecated from v1.1.0 |
# Query vehicle information
bluetoothKit.queryVehicleInformation(new OnQueryVehicleInfoListener() {
@Override
public void onQueryVehicleInfoSuccess(QueryVehicleInformation queryVehicleInformation) {
Log.d("bluetoothKit","QueryVehicleInfoSuccess");
}
@Override
public void onQueryVehicleInfoFail(int code, String msg) {
Log.e("bluetoothKit","QueryVehicleInfoFail");
}
});
attached:'QueryVehicleInformation'
field | type | description | note |
---|---|---|---|
powerPercent | int | battery percentage, 80->80% | supported from v1.1.0 |
speedMode | int | speed mode, 1: low speed; 2: medium speed; 3: high speed | supported from v1.1.0 |
currentSpeed | int | current speed, unit: km/h | |
totalRange | int | total range, unit: 10m | supported from v1.1.0 |
remainingRange | int | remaining range, unit: 10m | supported from v1.1.0 |
currentBatteryLevel | int | use `powerPercent` instead | deprecated from v1.1.0 |
currentSpeed | int | use `speedMode` instead | deprecated from v1.1.0 |
singleMileage | int | use `totalRange` instead | deprecated from v1.1.0 |
range | int | use `remainingRange` instead | deprecated from v1.1.0 |
# Open the battery cover
bluetoothKit.openBatteryCover(new OnOpenBatteryCoverListener() {
@Override
public void OnOpenBatteryCoverSuccess() {
Log.d("bluetoothKit","OpenBatteryCoverSuccess");
}
@Override
public void OnOpenBatteryCoverFail(int code, String msg) {
Log.e("bluetoothKit","OpenBatteryCoverFail");
}
});
# Open the saddle
bluetoothKit.openSaddle(new OnOpenSaddleListener() {
@Override
public void onOpenSaddleSuccess() {
Log.d("bluetoothKit","OpenSaddleSuccess");
}
@Override
public void onOpenSaddleFail(int code, String msg) {
Log.e("bluetoothKit","OpenSaddleFail");
}
});
# Open the tailBox
bluetoothKit.openTailBox(new OnOpenTailBoxListener() {
@Override
public void onOpenTailBoxSuccess() {
Log.d("bluetoothKit","OpenTailBoxSuccess");
}
@Override
public void onOpenTailBoxFail(int code, String msg) {
Log.e("bluetoothKit","OpenTailBoxFail");
}
});
# Unlock helmet supported from v1.1.2 support pre-installed helmet lock only
bluetoothKit.unLock(new OnUnlockHelmetListener() {
@Override
public void onUnlockHelmetSuccess() {
Log.d("bluetoothKit","UnlockHelmetSuccess");
}
@Override
public void onUnlockHelmetFail(int code, String msg) {
Log.e("bluetoothKit","UnlockHelmetFail");
}
});
# Lock helmet supported from v1.1.2 support pre-installed helmet lock only
bluetoothKit.lockHelmet(new OnLockHelmetListener() {
@Override
public void onLockHelmetSuccess() {
Log.d("bluetoothKit","LockHelmetSuccess");
}
@Override
public void onLockHelmetFail(int code, String msg) {
Log.d("bluetoothKit","LockHelmetFail");
}
});
# Helmet API Guide
# Init supported from v1.1.0
WARNING
Before calling this method, you need to init the NBIotBle in your application, otherwise you will not get the relative permission.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//init HelmetLockKit
HelmetLockKit helmetLockKit = new HelmetLockKit();
helmetLockKit.init(this);
}
# Connect supported from v1.1.0
Connect the device with the MAC Address and deviceKey, make sure the helmet is not connected to another bluetooth device before connecting.
private void connect() {
helmetLockKit.connect("deviceMac", "deviceKey", new OnConnectionStateChangeListener() {
@Override
public void onConnectionStateChange(int state) {
switch (state) {
case ConnectionState.STATE_CONNECTED:
Log.d("NBBleSDK","Connected");
break;
case ConnectionState.STATE_DISCONNECTED:
Log.d("NBBleSDK","Disconnected");
break;
default:
Log.d("NBBleSDK","ConnectFailed");
break;
}
}
});
}
# Disconnect supported from v1.1.0
Disconnect from a device
if (helmetLockKit != null) {
helmetLockKit.disConnect();
}
# Unlock helmet supported from v1.1.0
helmetLockKit.unLock(new OnUnlockHelmetListener() {
@Override
public void onUnlockHelmetSuccess() {
Log.d("helmetLockKit","UnlockSuccess");
}
@Override
public void onUnlockHelmetFail(int code, String msg) {
Log.e("helmetLockKit","UnlockFail");
}
});
# Query helmet status supported from v1.1.0
helmetLockKit.queryLockStatus(new OnQueryHelmetLockStatusListener() {
@Override
public void onQueryHelmetLockStatusSuccess(HelmetLockStatus helmetLockStatus) {
Log.d("helmetLockKit","onQueryHelmetLockStatusSuccess");
}
@Override
public void onQueryHelmetLockStatusFail(int code, String msg) {
Log.e("helmetLockKit","onQueryHelmetLockStatusFail");
}
});
attached:'HelmetLockStatus'
field | type | description | note |
---|---|---|---|
isLocked | boolean | true = locked,false = unlocked | |
voltage | int | current voltage of IoT, unit: mV |
# Query helmet information supported from v1.1.0
helmetLockKit.queryLockInfo(new OnQueryHelmetLockInfoListener() {
@Override
public void onQueryHelmetLockInfoSuccess(HelmetLockInformation helmetLockInformation) {
Log.d("helmetLockKit","onQueryHelmetLockInfoSuccess");
}
@Override
public void onQueryHelmetLockInfoFail(int code, String msg) {
Log.d("helmetLockKit","onQueryHelmetLockInfoFail");
}
});
attached:'HelmetLockInfo'
field | type | description | note |
---|---|---|---|
powerPercent | int | battery percentage, 80->80% | |
firmwareVersion | String | firmware version |
# Release Note
# v1.1.2
- Add interface of lock helmet for the new device of Helmet & Cable lock
# v1.1.1
- Optimized the device connection process by the reconnection attempts
# v1.1.0
- Add status of lock in the interface of Query IoT information
- Add field description in the query result
- Add support for helmet lock
# v1.0.0
- initial version